Remark : uAPI Soap Call and parsing sample java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
import javax.xml.soap.*; import java.io.*; import java.util.Base64; public class SoapConsole { public static void main(String[] args) { // TODO Auto-generated method stub String soapEndpointUrl = "https://apac.universal-api.pp.travelport.com/B2BGateway/connect/uAPI/AirService"; //요금조회서비스 String reqEnv = "<soapenv:Envelope xmlns:soapenv = 'http://schemas.xmlsoap.org/soap/envelope/'" + " xmlns:air='http://www.travelport.com/schema/air_v50_0' " + " xmlns:com='http://www.travelport.com/schema/common_v50_0'>" + " <soapenv:Body>" + " <air:LowFareSearchReq TraceId='2021122918322322' TargetBranch='P*******' SolutionResult='true' AuthorizedBy='user' >" + " <com:BillingPointOfSaleInfo OriginApplication='UAPI'/>" + " <air:SearchAirLeg>" + " <air:SearchOrigin>" + " <com:CityOrAirport Code='DEL'/>" + " </air:SearchOrigin>" + " <air:SearchDestination>" + " <com:CityOrAirport Code='BOM'/>" + " </air:SearchDestination>" + " <air:SearchDepTime PreferredTime='2022-05-12'> </air:SearchDepTime>" + " </air:SearchAirLeg>" + " <air:AirSearchModifiers>" + " <air:PreferredProviders>" + " <com:Provider Code='1G' />" + " </air:PreferredProviders>" + " <air:PermittedCarriers>" + " <com:Carrier Code='AI' />" + " </air:PermittedCarriers>" + " <air:PreferredCabins>" + " <com:CabinClass Type='Economy' />" + " </air:PreferredCabins>" + " <air:FlightType NonStopDirects='true' StopDirects='true' SingleOnlineCon='true' DoubleOnlineCon='false' TripleOnlineCon='false' SingleInterlineCon='false' DoubleInterlineCon='false' TripleInterlineCon='false' />" + " </air:AirSearchModifiers>" + " <com:SearchPassenger Code='ADT'/>" + " <air:AirPricingModifiers ETicketability='Required' FaresIndicator='AllFares'/>" + " </air:LowFareSearchReq>" + " </soapenv:Body>" + "</soapenv:Envelope>"; callSoapWebService(soapEndpointUrl, reqEnv); //System.out.println("Hello Java"); } private static void callSoapWebService(String soapEndpointUrl, String reqEnv) { try { // Create SOAP Connection SOAPConnectionFactory soapConnectionFactory = SOAPConnectionFactory.newInstance(); SOAPConnection soapConnection = soapConnectionFactory.createConnection(); MessageFactory msgFactory = MessageFactory.newInstance(); SOAPMessage reqMsg = msgFactory.createMessage(null, new ByteArrayInputStream(reqEnv.getBytes())); String name = "Put your Username received in Welcome letter here"; String password = "Put your password in Welcome letter here"; String authString = name + ":" + password; String encodedString = Base64.getEncoder().encodeToString(authString.getBytes()); reqMsg.getMimeHeaders().addHeader("Authorization", "Basic " + encodedString); SOAPMessage soapResponse = soapConnection.call(reqMsg, soapEndpointUrl); // Print the SOAP Response System.out.println("Response SOAP Message:"); soapResponse.writeTo(System.out); System.out.println(); soapConnection.close(); } catch (Exception e) { System.err.println("\nError occurred while sending SOAP Request to Server!\nMake sure you have the correct endpoint URL and SOAPAction!\n"); e.printStackTrace(); } } } |
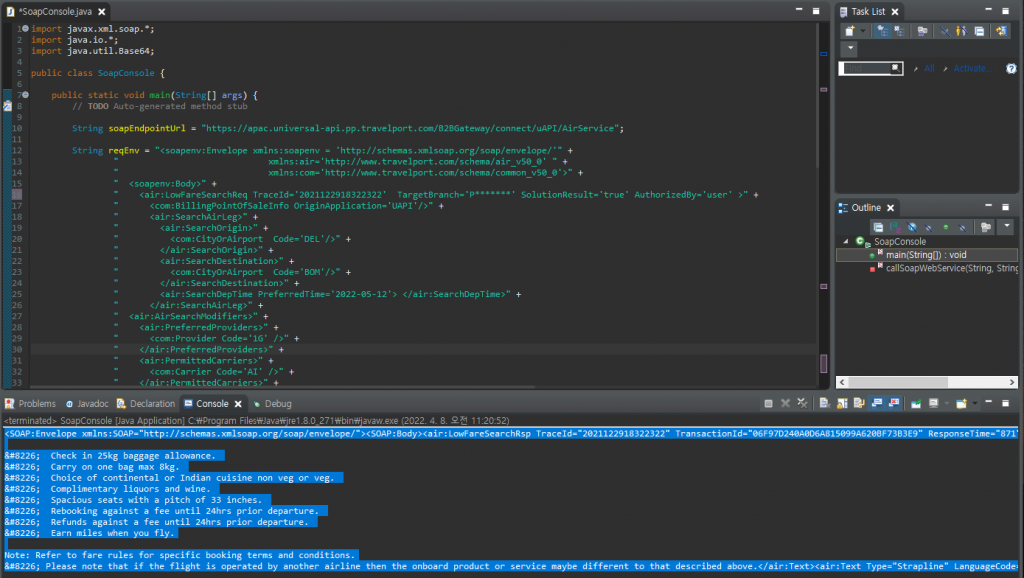